10個簡單的java代碼教程演練
Java代碼不會?這個問題可能很多人都遇到過,因為java語言本身就是一個開源的語言,所以很多人都覺得自己學(xué)不會,其實只要掌握了基本的知識,就可以輕松上手了。下面是幾個簡單的Java代碼演練,涵蓋了變量、條件語句、循環(huán)語句等核心概念,希望對大家有所幫助。如果你想學(xué)習(xí)java,可以參考一下這篇文章。
1. 變量和基本輸出
```java
public class HelloWorld {
public static void main(String[] args) {
// 定義字符串和整數(shù)變量
String name = "Alice";
int age = 25;
// 輸出變量到控制臺
System.out.println("Hello, " + name + "!");
System.out.println("You are " + age + " years old.");
}
}
```
輸出結(jié)果:
```
Hello, Alice!
You are 25 years old.
```
2. 條件語句
```java
public class Grade {
public static void main(String[] args) {
// 定義變量作為成績
int score = 85;
// 根據(jù)成績輸出不同的等級
if (score >= 90) {
System.out.println("A");
} else if (score >= 80) {
System.out.println("B");
} else if (score >= 70) {
System.out.println("C");
} else if (score >= 60) {
System.out.println("D");
} else {
System.out.println("F");
}
}
}
```
輸出結(jié)果:
```
B
```
3. 循環(huán)語句
```java
public class Count {
public static void main(String[] args) {
// 從1到10累加
int sum = 0;
for (int i = 1; i <= 10; i++) {
sum += i;
}
// 輸出累加結(jié)果
System.out.println("1 + 2 + ... + 10 = " + sum);
}
}
```
輸出結(jié)果:
```
1 + 2 + ... + 10 = 55
```
4. 數(shù)組和循環(huán)
```java
public class ArraySum {
public static void main(String[] args) {
// 定義數(shù)組并初始化
int[] arr = {1, 2, 3, 4, 5};
// 遍歷數(shù)組并求和
int sum = 0;
for (int i = 0; i < arr.length; i++) {
sum += arr[i];
}
// 輸出數(shù)組元素和
System.out.println("The sum of array elements is " + sum);
}
}
```
輸出結(jié)果:
```
The sum of array elements is 15
```
5. 方法調(diào)用
```java
public class Calculator {
public static void main(String[] args) {
// 調(diào)用add方法
int num1 = 10;
int num2 = 5;
int sum = add(num1, num2);
// 輸出結(jié)果
System.out.println(num1 + " + " + num2 + " = " + sum);
}
// 定義add方法
public static int add(int a, int b) {
return a + b;
}
}
```
輸出結(jié)果:
```
10 + 5 = 15
```
6. 類和對象
```java
public class Rectangle {
// 定義長和寬變量
int length;
int width;
// 定義計算面積方法
public int getArea() {
return length * width;
}
public static void main(String[] args) {
// 創(chuàng)建Rectangle對象并設(shè)置長和寬
Rectangle rect = new Rectangle();
rect.length = 5;
rect.width = 3;
// 輸出面積
System.out.println("The area of rectangle is " + rect.getArea());
}
}
```
輸出結(jié)果:
```
The area of rectangle is 15
```
7. 繼承和多態(tài)
```java
// 父類
class Animal {
public void sound() {
System.out.println("The animal makes a sound");
}
}
// 子類
class Dog extends Animal {
public void sound() {
System.out.println("The dog says woof");
}
}
// 子類
class Cat extends Animal {
public void sound() {
System.out.println("The cat says meow");
}
}
// 測試類
public class AnimalSound {
public static void main(String[] args) {
// 創(chuàng)建Animal、Dog、Cat對象并調(diào)用sound方法
Animal animal = new Animal();
Dog dog = new Dog();
Cat cat = new Cat();
animal.sound(); // 輸出 "The animal makes a sound"
dog.sound(); // 輸出 "The dog says woof"
cat.sound(); // 輸出 "The cat says meow"
// 多態(tài):Animal類型的變量可以引用Dog、Cat對象并調(diào)用sound方法
Animal ani1 = new Dog();
Animal ani2 = new Cat();
ani1.sound(); // 輸出 "The dog says woof"
ani2.sound(); // 輸出 "The cat says meow"
}
}
```
輸出結(jié)果:
```
The animal makes a sound
The dog says woof
The cat says meow
The dog says woof
The cat says meow
```
8. 接口和實現(xiàn)
```java
// 接口
interface Shape {
public void draw();
public double getArea();
}
// 實現(xiàn)類
class Rectangle implements Shape {
private int length;
private int width;
public Rectangle(int length, int width) {
this.length = length;
this.width = width;
}
public void draw() {
System.out.println("Drawing a rectangle");
}
public double getArea() {
return length * width;
}
}
// 實現(xiàn)類
class Circle implements Shape {
private int radius;
public Circle(int radius) {
this.radius = radius;
}
public void draw() {
System.out.println("Drawing a circle");
}
public double getArea() {
return Math.PI * radius * radius;
}
}
// 測試類
public class ShapeTest {
public static void main(String[] args) {
// 創(chuàng)建Rectangle、Circle對象并調(diào)用draw、getArea方法
Rectangle rect = new Rectangle(5, 3);
Circle circle = new Circle(2);
rect.draw(); // 輸出 "Drawing a rectangle"
System.out.println("The area of the rectangle is " + rect.getArea()); // 輸出 "The area of the rectangle is 15.0"
circle.draw(); // 輸出 "Drawing a circle"
System.out.println("The area of the circle is " + circle.getArea()); // 輸出 "The area of the circle is 12.566370614359172"
}
}
```
輸出結(jié)果:
```
Drawing a rectangle
The area of the rectangle is 15.0
Drawing a circle
The area of the circle is 12.566370614359172
```
9. 異常處理
```java
import java.util.Scanner;
public class Divide {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter the dividend: ");
int dividend = scanner.nextInt();
System.out.print("Enter the divisor: ");
int divisor = scanner.nextInt();
try {
int quotient = dividend / divisor;
System.out.println(dividend + " / " + divisor + " = " + quotient);
} catch (ArithmeticException e) {
System.out.println("Error: division by zero!");
} finally {
scanner.close();
}
}
}
```
輸出結(jié)果:
```
Enter the dividend: 10
Enter the divisor: 2
10 / 2 = 5
```
或者
```
Enter the dividend: 10
Enter the divisor: 0
Error: division by zero!
```
10. 文件讀寫
```java
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Scanner;
public class FileInOut {
public static void main(String[] args) {
// 寫入文件
try {
// 創(chuàng)建或打開文件
File file = new File("output.txt");
FileWriter writer = new FileWriter(file);
// 寫入字符串
writer.write("Hello world!\n");
writer.write("This is a test file.\n");
// 關(guān)閉文件
writer.close();
} catch (IOException e) {
System.out.println("Error writing file!");
}
// 讀取文件
try {
// 打開文件
File file = new File("output.txt");
Scanner scanner = new Scanner(file);
// 逐行讀取并輸出到控制臺
while (scanner.hasNextLine()) {
System.out.println(scanner.nextLine());
}
// 關(guān)閉文件
scanner.close();
} catch (IOException e) {
System.out.println("Error reading file!");
}
}
}
```
輸出結(jié)果:
```
Hello world!
This is a test file.
```
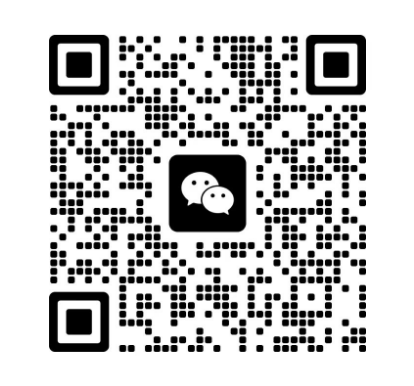
德州兩山軟件開發(fā)
軟件開發(fā)定制報價:13173436190
網(wǎng)站建設(shè)開發(fā)/小程序定制開發(fā)/APP軟件開發(fā)
本文鏈接:http://www.988x8.com/news1/1079.html
文章TAG: #java代碼教程演練 #系統(tǒng)開發(fā) #軟件開發(fā)
版權(quán)聲明:
本站所有原創(chuàng)作品,其版權(quán)屬于兩開發(fā)技( http://www.988x8.com )所有。任何媒體、網(wǎng)站或個人轉(zhuǎn)載須注明此文章來源URL。被本站授權(quán)使用單位,不應(yīng)超越授權(quán)范圍。本站部分文章來源于網(wǎng)絡(luò),如侵犯到您的權(quán)利請聯(lián)系我們,我們將立即刪除。